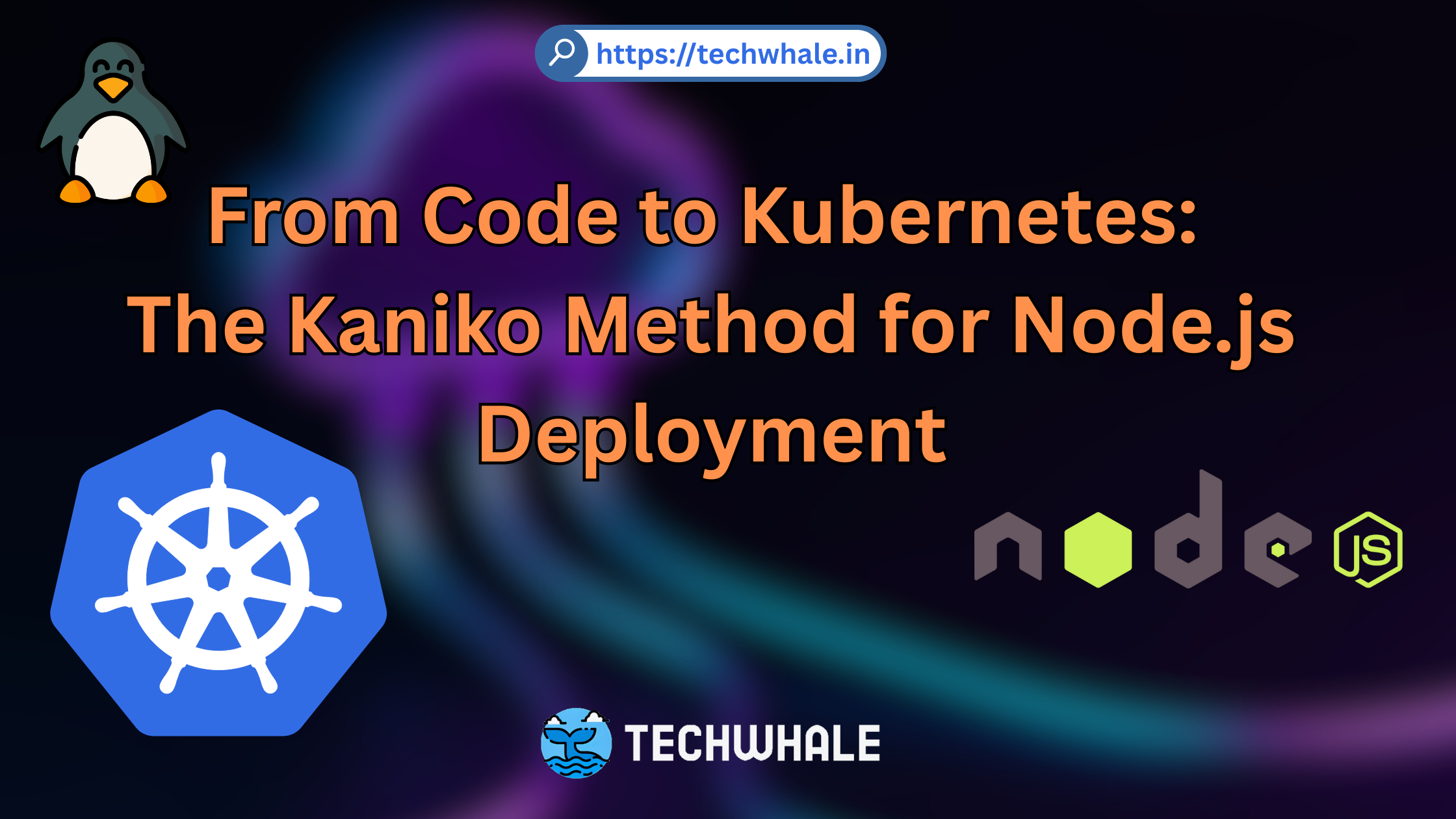
From Code to Kubernetes: The Kaniko Method for Node.js Deployment
Tired of wrestling with Docker-in-Docker for your Kubernetes deployments? Want to build container images right inside your cluster without the security headaches? This guide will walk you through deploying a Node.js application to Kubernetes using Kaniko - the container builder that works without needing a Docker daemon. Ready to level-up your DevOps skills? Let’s dive in! 🚀
Why This Matters (For Real-World Projects)
In modern development environments, building container images inside Kubernetes clusters has become increasingly necessary. But running Docker inside containers creates security vulnerabilities and permission nightmares.
Kaniko (think of it as your robot assistant that builds containers without the usual Docker overhead) solves this problem elegantly. Instead of requiring the Docker daemon, it executes container image builds in a standard Kubernetes pod, making your CI/CD pipeline more secure and flexible.
For production teams, this means:
- No more privileged containers just to build images
- Streamlined build process inside your existing Kubernetes infrastructure
- More consistent deployments across development and production
For solo developers, you’ll save countless hours debugging permission issues while keeping your deployment process clean and reproducible. It’s like having training wheels that actually make your bike faster! 🏎️💨
Setting Up Your Node.js App for Kaniko Deployment
1. Project Setup
First, let’s create a simple Node.js application that we’ll deploy:
mkdir k8s-node-kaniko-demo
cd k8s-node-kaniko-demo
npm init -y
npm install express
touch server.js Dockerfile build.yaml deployment.yaml
For our server.js file, we’ll create a super-simple Express server:
const express = require("express");
const app = express();
const PORT = process.env.PORT || 8080;
app.get("/", (req, res) => {
res.send(
"Hey there, Kubernetes explorer!Your Node.js app is running in a container built with Kaniko. Pretty cool, right?"
);
});
app.listen(PORT, () => {
console.log(`Server rocking and rolling on port ${PORT}`);
});
2. Creating Your Dockerfile
Now, let’s package our app with a straightforward Dockerfile:
# Base image - like picking the foundation for your house
FROM node:16-alpine
# Set up our workspace - like cleaning your room before starting homework
WORKDIR /app
# Copy package files first (this helps with caching)
COPY package*.json ./
# Install dependencies - like getting all ingredients before cooking
RUN npm install
# Copy the rest of the app
COPY . .
# Tell the world which port we're using
EXPOSE 8080
# Start the app - it's showtime!
CMD ["node", "server.js"]
3. Creating the Kaniko Build Configuration
Here’s where the Kaniko magic happens. Create a build.yaml file:
apiVersion: batch/v1
kind: Job
metadata:
name: kaniko-node-builder
spec:
template:
spec:
containers:
- name: kaniko
image: gcr.io/kaniko-project/executor:latest
args:
- "--dockerfile=Dockerfile"
- "--context=git://github.com/YOUR_USERNAME/k8s-node-kaniko-demo.git#refs/heads/main"
- "--destination=YOUR_DOCKERHUB_USERNAME/node-kaniko-demo:latest"
volumeMounts:
- name: docker-config
mountPath: /kaniko/.docker/config.json
subPath: config.json
restartPolicy: Never
volumes:
- name: docker-config
secret:
secretName: docker-credentials
4. Setting Up Kubernetes Deployment
Create your deployment.yaml file to run the app in Kubernetes:
apiVersion: apps/v1
kind: Deployment
metadata:
name: node-kaniko-app
spec:
replicas: 2
selector:
matchLabels:
app: node-kaniko-app
template:
metadata:
labels:
app: node-kaniko-app
spec:
containers:
- name: node-app
image: YOUR_DOCKERHUB_USERNAME/node-kaniko-demo:latest
ports:
- containerPort: 8080
---
apiVersion: v1
kind: Service
metadata:
name: node-app-service
spec:
selector:
app: node-kaniko-app
ports:
- port: 80
targetPort: 8080
type: LoadBalancer
Let’s Deploy This Thing!
Now that our files are ready, let’s execute our plan step by step:
1. Push Your Code to GitHub
git init
git add .
git commit -m "Initial commit with Node.js app for Kaniko deployment"
git remote add origin https://github.com/YOUR_USERNAME/k8s-node-kaniko-demo.git
git push -u origin main
2. Create Docker Credentials Secret
Before running Kaniko, we need to set up a secret for Docker Hub authentication:
kubectl create secret docker-registry docker-credentials \
--docker-username=YOUR_DOCKERHUB_USERNAME \
--docker-password=YOUR_DOCKERHUB_PASSWORD \
--docker-email=YOUR_EMAIL
3. Run the Kaniko Build Job
kubectl apply -f build.yaml
Watch the build progress:
kubectl get pods
kubectl logs -f $(kubectl get pods -l job-name=kaniko-node-builder -o jsonpath='{.items[0].metadata.name}')
4. Deploy Your Application
Once the image is built and pushed:
kubectl apply -f deployment.yaml
5. Access Your Application
kubectl get services node-app-service
Look for the EXTERNAL-IP and access your app at http://ip.
.
Pro Tips for Kaniko Kubernetes Masters ⚠️
⚠️ Context Is Everything
When specifying the Git context in build.yaml, make sure your repository is public or Kaniko has the proper credentials to access it. Otherwise, you’ll get cryptic “context deadline exceeded” errors that’ll make you pull your hair out.
⚠️ Cache Those Layers
Want to speed up your builds? Add the --cache=true
argument to Kaniko to enable layer caching:
args:
- "--dockerfile=Dockerfile"
- "--context=git://github.com/..."
- "--destination=..."
- "--cache=true"
⚠️ Always Check Your Registry Credentials
If your Kaniko builds fail with “unauthorized: authentication required” errors, your Docker Hub credentials are likely incorrect or expired. Don’t waste hours debugging - check those first!
# Test your credentials work
kubectl get secret docker-credentials -o jsonpath='{.data.\.dockerconfigjson}' | base64 -d
In very simple language: What’s Really Going On Here?
Kubernetes (like a super-organized lunch table coordinator at school): It’s a system that manages where and how your app containers run, making sure they’re healthy and accessible.
Kaniko (like building LEGO models without the official LEGO factory): A tool that builds container images without needing Docker installed - it works right inside Kubernetes!
Node.js app (like your school project that needs to be presented to the class): Your JavaScript code that needs to be packaged up so it can run anywhere.
Container image (like a perfectly packed lunch box): A standardized package containing your application and everything it needs to run.
Docker Hub (like Instagram for your lunch boxes): A place where you store your container images so Kubernetes can download and run them.
Troubleshooting When Things Go Sideways
If y’all run into issues (and let’s be honest, we all do), here are some common problems and solutions:
Kaniko job never completes: Check your git repository URL and make sure the repository is accessible.
Image builds but deployment fails: Verify your image name in the deployment file matches exactly what Kaniko pushed.
Can’t access your application: If your service shows `` for EXTERNAL-IP, your Kubernetes cluster might not support LoadBalancer services. Try using NodePort instead:
spec:
type: NodePort
ports:
- port: 80
targetPort: 8080
nodePort: 30080
Then access your app at http://ip:30080
.
Wrapping It Up
Congratulations! You’ve successfully deployed a Node.js application to Kubernetes using Kaniko. No more privileged containers or Docker-in-Docker headaches! This approach gives you a more secure and flexible way to build container images directly within your Kubernetes ecosystem.
Remember, DevOps isn’t just about following steps—it’s about understanding why each piece matters and how they all fit together to create a smooth deployment pipeline. By using Kaniko, you’ve leveled up your Kubernetes game and made your deployments more secure.
What other containerization tools would you like to try with Kubernetes? Let us know in the comments below! 🚀💡🔧